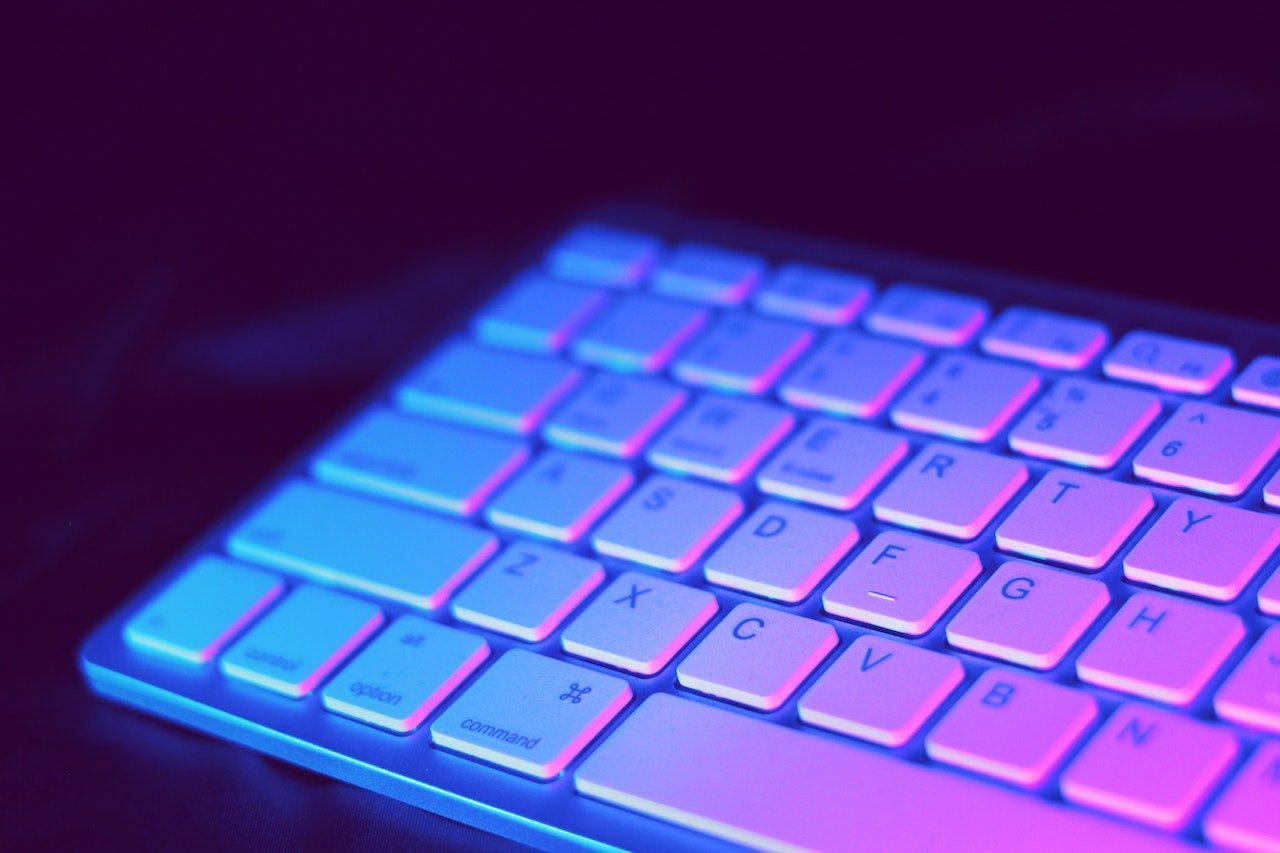
The Art of Laravel Framework Migrations and Upgrades
Introduction
In the realm of web development, Laravel has emerged as a powerful and popular PHP framework, known for its elegant syntax, robust features, and developer-friendly environment. One of the key components that make Laravel a standout framework is its migration and upgrade system. In this article, we will delve into the intricacies of Laravel framework migrations and upgrades, exploring the methods, best practices, and tools that developers can leverage to manage database schema changes effectively.
Understanding Laravel Migrations
At the core of Laravel's database management lies the concept of migrations. Migrations are essentially PHP files that allow developers to define and manage database schema changes in a systematic and version-controlled manner. By using migrations, developers can track and apply changes to the database structure without the need for manual SQL queries or manual intervention.
Creating Migrations
To create a new migration in Laravel, developers can use the `php artisan make:migration` command followed by the desired migration name. This command generates a new migration file in the `database/migrations` directory, where developers can define the schema changes they wish to make.
```php
php artisan make:migration create_users_table
```
Defining Schema Changes
Within a migration file, developers can use a variety of methods provided by Laravel's Schema Builder to define the desired database changes. For example, to create a new table, developers can use the `create` method and specify the table name and its columns.
```php
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->timestamps();
});
```
### Running Migrations
Once the migration file is created and the schema changes are defined, developers can run the migrations using the `php artisan migrate` command. This command applies the pending migration files to the database, creating or modifying the database schema according to the defined changes.
```php
php artisan migrate
```
Upgrading Database Schema in Laravel
As applications evolve and requirements change, developers often need to upgrade the database schema to accommodate new features or data structures. Laravel provides a seamless mechanism for upgrading database schema using migrations, allowing developers to make incremental changes to the database without disrupting the existing data.
Making Schema Changes
When upgrading the database schema in Laravel, developers can create new migration files to define the changes that need to be applied. Whether it's adding a new column, modifying an existing column, or creating an index, developers can use Laravel's Schema Builder methods within the migration files to make the necessary modifications.
```php
Schema::table('users', function (Blueprint $table) {
$table->string('username')->unique();
});
```
Applying Schema Upgrades
To apply the schema upgrades defined in the migration files, developers can run the `php artisan migrate` command. Laravel's migration system keeps track of the migrated files, ensuring that only the pending migrations are executed when the command is run.
```php
php artisan migrate
```
Rolling Back Migrations
In case an error occurs during a migration or if developers need to revert a schema change, Laravel provides the `php artisan migrate:rollback` command to roll back the last batch of migrations. This command effectively undoes the last set of migration operations, allowing developers to correct any issues or revert unwanted changes.
```php
php artisan migrate:rollback
```
Best Practices for Laravel Migrations and Upgrades
As with any development process, there are best practices that developers can follow to ensure smooth and efficient management of database schema changes in Laravel. By adhering to these practices, developers can maintain a clean and reliable database structure while minimizing the risk of errors and data loss.
Version Control
One of the fundamental principles of migrations and upgrades is version control. By keeping all migration files in the version control system, developers can track the evolution of the database schema over time, identify changes made by different team members, and roll back to previous versions if needed.
Atomic Migrations
To ensure consistency and reliability in database changes, developers should strive to make migrations atomic. Atomic migrations encapsulate a single logical change, such as creating a new table or adding a column, in a single migration file. This approach makes it easier to manage and track individual changes, reducing the risk of conflicts or errors.
Testing Migrations
Before running migrations in a production environment, developers should test the migration files in a staging or development environment to validate their correctness and compatibility with the application. By testing migrations thoroughly, developers can catch potential issues early and prevent data corruption or loss in the production database.
Rollback Strategy
In addition to rolling back individual migrations, developers should also plan for a comprehensive rollback strategy in case of a catastrophic failure or data corruption. By having a backup plan and a clear rollback procedure in place, developers can mitigate the impact of unexpected issues during the migration process.
Advanced Techniques in Laravel Migrations
While Laravel provides a robust set of tools and methods for managing database schema changes, there are advanced techniques that developers can employ to enhance the efficiency and flexibility of migrations.
Using Raw SQL Queries
Although Laravel's Schema Builder provides a convenient way to define schema changes using PHP methods, developers can also execute raw SQL queries within migration files for complex or specific database operations. By leveraging raw SQL queries, developers can take full control over the database interactions and execute custom commands as needed.
```php
DB::statement('ALTER TABLE users ADD COLUMN bio TEXT');
```
Custom Migration Templates
To streamline the migration process and enforce consistent coding standards, developers can create custom migration templates that include predefined schema structures, naming conventions, or default values. By using custom templates, developers can save time and ensure that migrations adhere to the project's guidelines.
Seed Data in Migrations
In addition to defining database schema changes, developers can also seed the database with initial data using migrations. By including data seeding logic within migration files, developers can populate the database with sample records or default values, making it easier to test the application's functionality and ensure that the database is initialized correctly.
```php
public function run()
{
DB::table('users')->insert([
'name' => 'John Doe',
'email' => 'john@example.com',
]);
}
```
Conclusion
In the dynamic world of web development, managing database schema changes is a critical aspect of building and maintaining robust applications. Laravel's migration and upgrade system provides developers with a powerful and flexible toolset to define, apply, and track database changes in a structured and controlled manner.
By understanding the principles of Laravel migrations, following best practices, and exploring advanced techniques, developers can leverage the full potential of Laravel's database management capabilities to create scalable, maintainable, and efficient applications.
Whether it's creating new tables, modifying columns, or upgrading the database schema, Laravel's migration system empowers developers to evolve their applications seamlessly while ensuring the integrity and consistency of the underlying database structure.
In the ever-evolving landscape of web development, mastering the art of Laravel framework migrations and upgrades is essential for developers looking to build modern, resilient, and high-performing applications that stand the test of time.